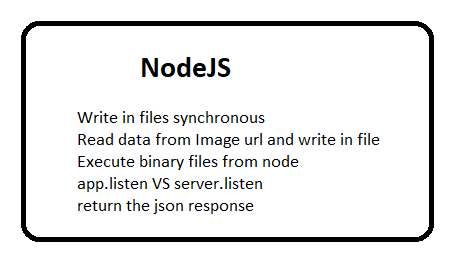
Question: How to Call async/await functions in parallel?
We can use await on Promise.all()
Syntax
Example
Question: How to read data from Image url and write in file synchronous?
First, We must need to install https and fs module in node.
https - Its for execute the https request.
fs - for save the file in local.
Example
Question: What is difference between app.listen and server.listen?
Both are used to start the server, for listening the request.
We need server.listen for create the request on https server.
app.listen will create http server.
Question: How to return the json response?
You can use res.json() to return the response in APIs
Example
Question: How to auto-reload files in Node.js in Local server?
While doing the development, you do not want to re-start the node server each time, when you made changes.
For this you can use nodemon module.
Install the nodemon module
start the node with nodemon instead of node
Question: How to create https request?
To Create the https server,
---first you need to install the modules like https, express and socket.io etc
---You need private/public keys for ssl
We can use await on Promise.all()
Syntax
let [someResult, anotherResult, anotherResult3] = await Promise.all([someCall(), anotherCall(), another3Call()]);
Example
const promise1 = Promise.resolve(3); const promise2 = 42; const promise3 = new Promise((resolve, reject) => { setTimeout(resolve, 100, 'foo'); }); Promise.all([promise1, promise2, promise3]).then((values) => { console.log(values); });
Question: How to read data from Image url and write in file synchronous?
First, We must need to install https and fs module in node.
https - Its for execute the https request.
fs - for save the file in local.
Example
const https = require('https'); const fs = require('fs'); const file = fs.createWriteStream("file.jpg");//Create a file const request = https.get("https://img.youtube.com/vi/0yX92mE6h2M/mqdefault.jpg", function(response) { response.pipe(file);//Add the image-content in file });
Question: What is difference between app.listen and server.listen?
Both are used to start the server, for listening the request.
We need server.listen for create the request on https server.
var https = require('https'); var server = https.createServer(app).listen(config.port, function() { console.log('Https App started'); });
app.listen will create http server.
var express = require('express'); var app = express(); app.listen(1234);
Question: How to return the json response?
You can use res.json() to return the response in APIs
Example
exports.get_data = function(req, res) { res.json({name: "web technology experts",description:"this is description"}); };
Question: How to auto-reload files in Node.js in Local server?
While doing the development, you do not want to re-start the node server each time, when you made changes.
For this you can use nodemon module.
Install the nodemon module
npm install nodemon -g
start the node with nodemon instead of node
nodemon app.js
Question: How to create https request?
To Create the https server,
---first you need to install the modules like https, express and socket.io etc
---You need private/public keys for ssl
var https = require('https'); var privateKey = fs.readFileSync('public/server.key', 'utf8'); var certificate = fs.readFileSync('public/file.crt', 'utf8'); var caCertificate = fs.readFileSync('public/chain.crt', 'utf8'); var credentials = {key: privateKey, cert: certificate, ca: caCertificate}; var httpsServer = https.createServer(credentials, app); var io = require('socket.io')(httpsServer); httpsServer.listen(443,function() { console.log('https listening on 443'); });