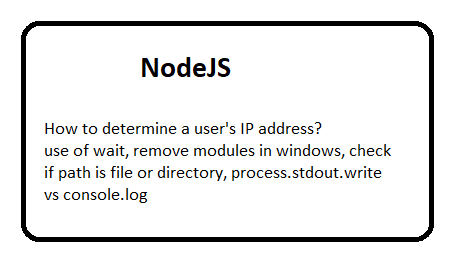
Question: How to determine a user's IP address in node?
We can use request.connection.remoteAddress to detect the IP Address in node but sometimes server is running behind the load balancer, In this case we need to check for x-forwarded-for
Example
exports.check_node = function(req, res) { var ip = req.headers['x-forwarded-for'] || req.connection.remoteAddress || req.socket.remoteAddress || (req.connection.socket ? req.connection.socket.remoteAddress : null); res.json({ip:ip}); };
Question: How can I use wait In Node.js?
You can use setTimeout function similar to javascript
Example
function function1() { console.log('Welcome to My Console,'); } function function2() { console.log('Console2'); } setTimeout(function2, 3000); //call second function1(); //Call first
Question: Command to remove all npm modules in windows?
- Remove module local
Go to directory with name of node_modules under current project. DELETE all the folder. -
C:\Users\DELL\AppData\Roaming\npm\node_modules
Delete all the files
Question: Node.js check if path is file or directory?
For this, you need to install the fs module.
var fs = require('fs'); stat = await fs.lstat(PATH_HERE) stats.isFile() stats.isDirectory() stats.isBlockDevice() stats.isCharacterDevice() stats.isSocket()
Question: What is use of app.use in express?
app.use is a method to configure the middleware used by Express.
Question: Difference between process.stdout.write and console.log?
console.log() calls process.stdout.write with formatted output.
console.log() explains in library
console.log = function (d) { process.stdout.write(d + '\n'); };
Question: How to Detect if file called through require or directly by command line?
if (require.main === module) { console.log('called directly'); } else { console.log('required as a module'); }
Question: What is the use of next() in express?
It passes control to the next matching route.
For Example:
app.get('/user/:id?', function(req, res, next){ var id = req.params.id; if (id) { // do something } else { next(); } });