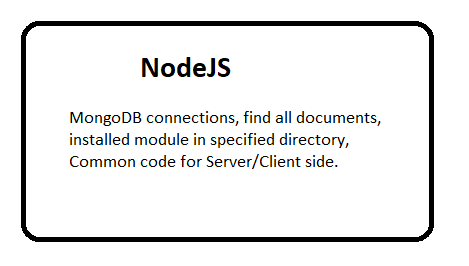
Question: How do I manage MongoDB connections in a Node.js web application?
You need to install mongoose and bluebird module.
Also you must have mongodb install in server, As you need mongodb URL and port on which mongodb running.
Example
var mongoose = require('mongoose'); mongoose.Promise = require('bluebird'); //mongodb connection with error handing mongoose.connect(config.MONGO_DB_URL + config.MONGO_DB); var db = mongoose.connection; db.on('error', console.error.bind(console, 'connection error:')); db.once('open', function() { console.log("MongoDB connected Successfully.!"); });
Question: mongoose - find all documents with IDs listed in array?
var AjaxChatUser = mongoose.model('AjaxChatUser'); AjaxChatUser.find({ '_id': { $in: [ mongoose.Types.ObjectId('4ed3ede8844f0f351100000c'), mongoose.Types.ObjectId('4ed3f117a844e0471100000d'), mongoose.Types.ObjectId('4ed3f18132f50c491100000e') ]} }, function(err, docs){ console.log(docs); });
Question: How to npm install to a specified directory?
Use --prefix option, to installed in specific directory.
Example
npm install --prefix-g
Question: How to declare multiple module.exports in Node.js?
You can put multiple function inside module.exports.
Example
module.exports = { method: function() {}, otherMethod: function() {}, };
Question: Can we write a JS code that work for both (node and the browser)?
Yes, We can write.
Suppose we have mymodule.js which have following code.
In Node (Server side()
In Browser (Client side()
Question: How we can use global variable in Node?
We can use with global.varname
Question: How we can access static files with express.js in Node?
Use following to set the folder as static so that we can put public files here.
Yes, We can write.
Suppose we have mymodule.js which have following code.
Example of Code (function(exports){ exports.test1 = function(){ return 'this is test1 function.' }; exports.test2 = function(){ return 'this is test2 function.' }; })(typeof exports === 'undefined'? this['mymodule']={}: exports);
In Node (Server side()
var share = require('./mymodule.js'); share.test1(); share.test2();
In Browser (Client side()
//Include the js with script tag share.test1(); share.test2();
Question: How we can use global variable in Node?
We can use with global.varname
global.version='1022.55';
Question: How we can access static files with express.js in Node?
Use following to set the folder as static so that we can put public files here.
app.use(express.static('public')); //public folder